I like to learn something new: new technologies, languages, countries, food, and experiences — all of that is a big part of my life’s joy.
During thinking about my next OKR goal with folks from JetBridge I came up with an idea for how to build something interesting which can help me learn new words and phrases in a more productive way.
The main idea sounds simple: to implement a with a Telegram app bot for learning new words using Anki periodic repetition algorithm. To make it more interesting (and complex 🙃) I decided that it needs to be serverless with AWS lambda and have infrastructure managed by pulumi. This article describes my approach.
The infrastructure contains:
- API Gateway to handle incoming requests and pass them forward
- Lambda to process user commands
- RDS Postgres database instance for storage

Pulumi allows managing code infrastructure in a convenient manner using any programming language. It’s great when you can define and configure the infrastructure using the same language as the app. In my case, it is Go.
Another benefit that pulumi provides out of the box is using variables directly from created resources. For example, you create an RDS Instance, and then an endpoint and DB properties are available to be used as lambda env variables. It means you don’t need to hard-code such variables or store them anywhere, pulumi manages them for you. Even in the case when some resource is changed (for example a major RDS upgrade will create a new instance) all related infrastructure will be up to date.
The bigger project would require a much more complex definition and resources stack, but for my purpose, it’s rather small and can be kept in only one file.
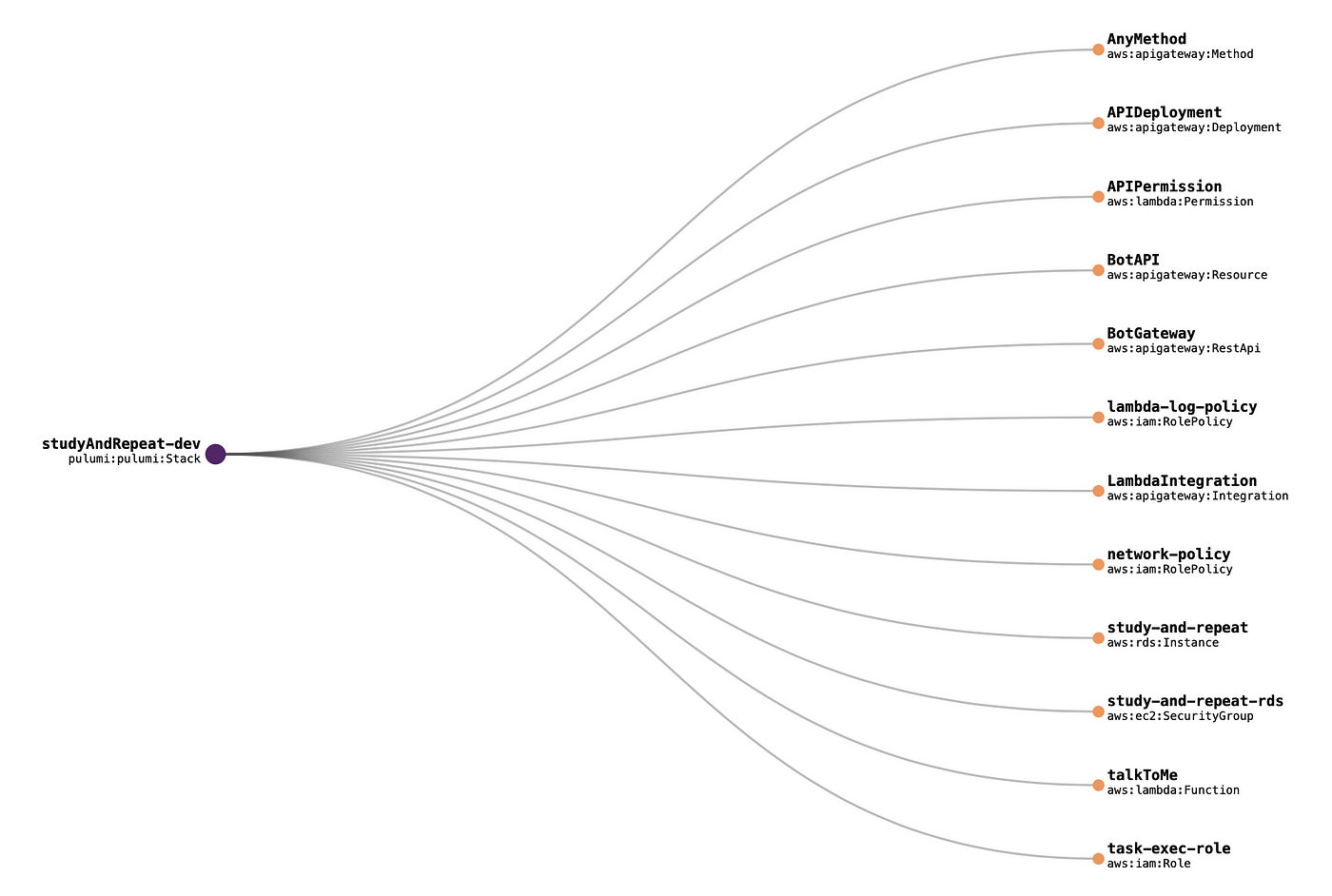
One more cool feature about pulumi is managing secrets. Usually, you need to configure and use some service for it (AWS Secret store, Vault, etc) but with Pulumi you can use it out of the box with a simple command:
pulumi config set --secret DB_PASSWORD <password>
And then use it as an environmental or any other specified property of your app. Additionally, you can configure a key for encryption which is stored in pulumi by default but can easily be changed to a key from AWS KMS or other available provider:
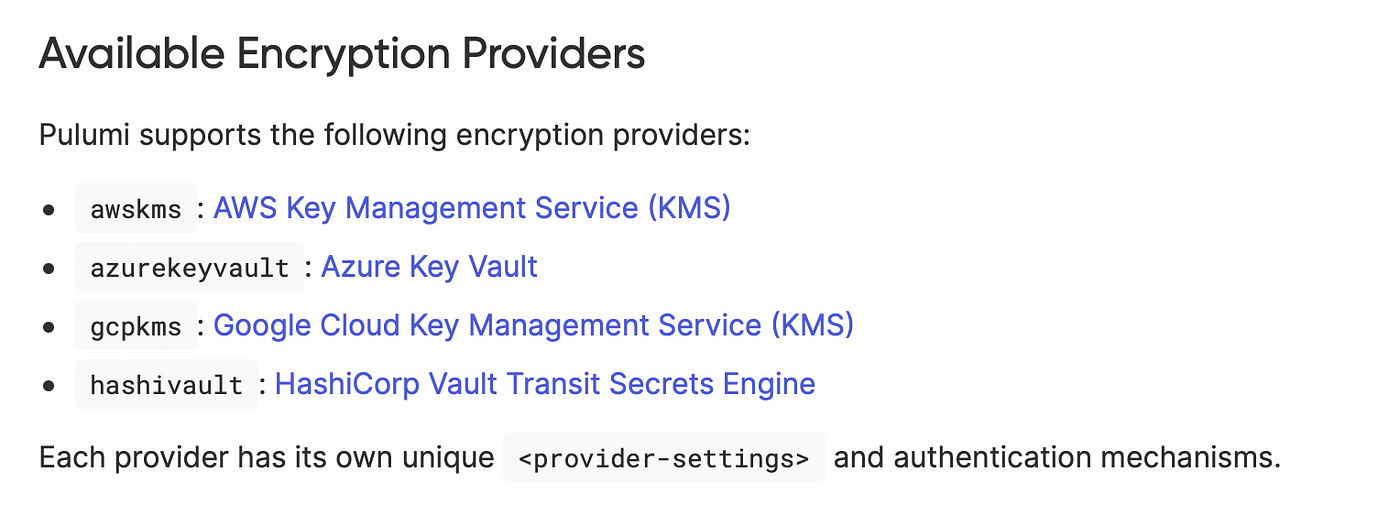
Exported properties allow us to see the most important infra params directly in the CLI or pulumi interface:
ctx.Export("DB Endpoint", pulumi.Sprintf("%s", db.Endpoint))

To deploy a lambda update, it needs to be built and archived. Then pulumi just sends this archive as a lambda source:
function, err := lambda.NewFunction(
ctx,
"talkToMe",
&lambda.FunctionArgs{
Handler: pulumi.String("handler"),
Role: role.Arn,
Runtime: pulumi.String("go1.x"),
Code: pulumi.NewFileArchive("./build/handler.zip"),
Environment: &lambda.FunctionEnvironmentArgs{
Variables: pulumi.StringMap{
"TG_TOKEN": c.RequireSecret("TG_TOKEN"),
"DB_PASSWORD": c.RequireSecret("DB_PASSWORD"),
"DB_USER": db.Username,
"DB_HOST": db.Address,
"DB_NAME": db.DbName,
},
},
},
pulumi.DependsOn([]pulumi.Resource{logPolicy, networkPolicy, db}),
)
To have it as a simple command I use a Makefile:
build::
GOOS=linux GOARCH=amd64 go build -o ./build/handler ./src/handler.go
zip -j ./build/handler.zip ./build/handler
Then I can trigger deploy just with make build && pulumi up -y
After that pulumi defines if the archive was changed and sends an update if so. For my simple project, it takes about 20s which is quite fast.
GOOS=linux GOARCH=amd64 go build -o ./build/handler ./src/handler.go
zip -j ./build/handler.zip ./build/handler
updating: handler (deflated 45%)
View Live: https://app.pulumi.com/<pulumi-url>
Type Name Status Info
pulumi:pulumi:Stack studyAndRepeat-dev
~ └─ aws:lambda:Function talkToMe updated (12s) [diff: ~code]
Outputs:
DB Endpoint : "<endpoint>.eu-west-1.rds.amazonaws.com:5432"
Invocation URL: "https://<endpoint>.execute-api.eu-west-1.amazonaws.com/dev/{message}"
Resources:
~ 1 updated
12 unchanged
Duration: 20s
Here you can take a look at the whole infrastructure code.
In conclusion, I can say that pulumi is a great tool to write and keep infrastructure structured, consistent and readable. I used CloudFormation and Terraform previously and pulumi gives me a more user-friendly and pleasant way to have infrastructure as code.